※この記事は「2021年4月15日」に更新しました。
JavaScript講座、タイピングゲームを作成する No.4 です。
前回の記事を確認したい方は、下記からどうぞ。
今回は、ゲームをスタートするときのイベントやタイマー処理を追加していきます。
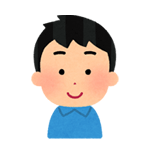
タイピングゲームを作成する
それでは、タイピングゲームを作成します。
今回の記事のポイントは、以下の通りです。
- ゲームをスタートするときのイベント
- タイマー処理
それでは、順番に解説していきます。
ゲームをスタートするときのイベント
ゲームをスタートするときのイベントを追加していきます。
まず、No.1 で紹介した HTML のコードを改良します。
<html lang="ja"> <head> <meta charset="utf-8"> <title>ぱそたのタイピング道場</title> <link rel="stylesheet" href="css/style.css" </head> <body> <h1>My Typing Game</h1> <hr> <p id="typingWord"> Please click </p> <hr> <p id="info"> 入力文字数:<span id="wordCount">0</span> タイプミス:<span id="typeMiss">0</span> 残り時間:<span id="timeLeft">0</span> </p> <script src="js/main.js"></script> </body> </html>
typingWord 部分の内容は、最初に表示させるテキストを入力しています(Please click)。
次に JavaScript のコードです。
前回のサンプルコードである、以下のコード。
typingWord.textContent = word;
この部分を削除して、クリックするとタイプするワードを配置するという処理を追加します。
window.addEventListener('click', () => { typingWord.textContent = word; });
タイマー処理
続いて、タイマー処理を追加します。
早速、追加した JavaScript のサンプルコードを紹介します。
window.addEventListener('click', () => { typingWord.textContent = word; startTime = Date.now(); updateTimeLimit(); });
updateTimeLimit関数は、下記の通りです。
function updateTimeLimit() { const timer = startTime + timeLimit - Date.now(); timeLeftLabel.textContent = (timer / 1000).toFixed(2); const timeoutId = setTimeout(() => { updateTimeLimit(); }, 10); if (timer < 0) { clearTimeout(timeoutId); timeLeftLabel.textContent = '0.00'; setTimeout(() => { alert('Game Over'); }, 100); } }
ブラウザでこんなことができるのかと感心したのですが、動的にタイマー処理をして、制限時間がきたら、アラートが表示されるようになっています。
サンプルコード【JavaScript】
サンプルコードです(JavaScript)。
前回と同様 use strict 宣言だけ先頭に追記してください。
const words = [ 'Thomas', 'Edward', 'Henry', 'Gordon', 'James', 'Percy', 'Toby', ]; let word = words[Math.floor(Math.random() * words.length)]; let loc = 0; let wordCount = 0; let typeMiss = 0; const timeLimit = 60 * 1000; let startTime; const typingWord = document.getElementById('typingWord'); const wordCountLabel = document.getElementById('wordCount'); const typeMissLabel = document.getElementById('typeMiss'); const timeLeftLabel = document.getElementById('timeLeft'); function updateTypingWord() { let update = ''; for (let i = 0; i < loc; i++){ update += '-'; } typingWord.textContent = update + word.substring(loc); } function updateTimeLimit() { const timer = startTime + timeLimit - Date.now(); timeLeftLabel.textContent = (timer / 1000).toFixed(2); const timeoutId = setTimeout(() => { updateTimeLimit(); }, 10); if (timer < 0) { clearTimeout(timeoutId); timeLeftLabel.textContent = '0.00'; setTimeout(() => { alert('Game Over'); }, 100); } } window.addEventListener('click', () => { typingWord.textContent = word; startTime = Date.now(); updateTimeLimit(); }); window.addEventListener('keydown', e => { console.log(e.key); if (e.key === word[loc]){ loc++; if (loc === word.length) { word = words[Math.floor(Math.random() * words.length)]; loc = 0; } updateTypingWord(); wordCount++; wordCountLabel.textContent = wordCount; } else if (e.key === 'Shift') { ; } else { typeMiss++; typeMissLabel.textContent = typeMiss; } });
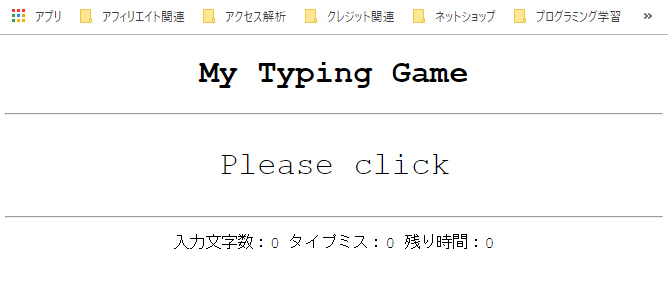
最後に
いかがでしょうか。
まだ、リプレイができないのと、ゲーム中でもクリックするとタイマーがリセットされてしまいます。
次回、修正していきたいと思います。