前回は、JavaScript の基礎文法について紹介しました。
今回は、関数について紹介していきます。
関数について
関数は、様々な処理をまとめて、名前をつけることができるものです。
同じ処理を何度も実行する場合、関数にしておけば、同じことを何度も書く必要がなくなり、修正するときも便利です。
関数を宣言して呼び出す(引数なし)
関数を宣言するには、function を使います。
例えば、メッセージを表示させる関数を適当につくります。
function message(){ console.log('Thomas!'); console.log('Edward!'); console.log('Henry!'); console.log('Gordon!'); console.log('James!'); console.log('Percy!'); console.log('Toby!'); }
次に定義した関数を呼び出しましょう。
message();
とすれば、先程定義した関数を呼び出すことができます。
結果は以下の通りです。
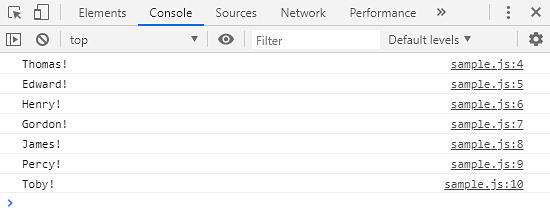
関数を宣言して呼び出す(引数あり)
関数に何らかの値を渡して、その値によって処理を変えたい場合、引数を使います。
例えば、1~7の数字によって表示させるメッセージを変化させる関数をつくります。
function message(number){ switch(number){ case 1: console.log('Thomas!'); break; case 2: console.log('Edward!'); break; case 3: console.log('Henry!'); break; case 4: console.log('Gordon!'); break; case 5: console.log('James!'); break; case 6: console.log('Percy!'); break; case 7: console.log('Toby!'); break; } } message(7); message(6); message(5); message(4); message(3); message(2); message(1);
結果は以下の通りです。
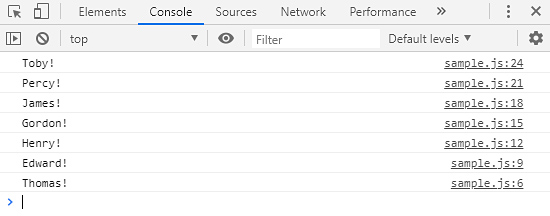
関数に戻り値を設定する
関数に戻り値を設定することで関数内で処理した結果を返すこともできます。
function message(number){ switch(number){ case 1: return 'Thomas!'; case 2: return 'Edward!'; case 3: return 'Henry!'; case 4: return 'Gordon!'; case 5: return 'James!'; case 6: return 'Percy!'; case 7: return 'Toby!'; } } console.log(message(1)); console.log(message(4));
結果は以下の通りです。
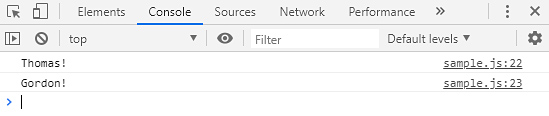
最後に
いかがでしょうか。
JavaScript でも関数を使うことができます。
ついでに前回紹介できなかった、switch文も適当に使ってみました。